FAQ
Frequently Asked Questions (FAQ)
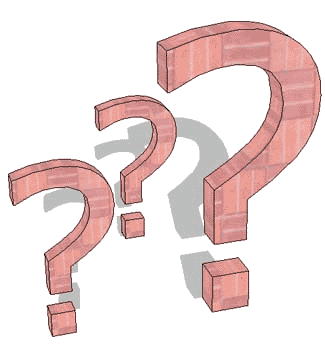
Basic Questions
What is SketchUp?
SketchUp is Trimble's easy-to-use 3d modeling software. It is available in both Make and Pro Versions from www.sketchup.com. It is used worldwide for drawing 3d objects of all kinds. You can find thousands of examples of what you can do with SketchUp at 3D Warehouse.What is Ruby?
Ruby is "an interpreted scripting language for quick and easy object-oriented programming." You can learn more at http://www.ruby-lang.org/. Most importantly for us, it's a language that SketchUp can understand via its SketchUp Ruby API.What is the SketchUp Ruby API?
The SketchUp Ruby API (Application Programming Interface) is a way that Ruby programmers can extend the capabilities of SketchUp to meet their needs. By creating a script (aka a text file) and placing it in SketchUp's plugins folder, you can make SketchUp do all kinds of things:- Create custom drawing tools, like a tool for creating windows.
- Attach attributes to drawing elements such as cost, supplier, etc.
- Read attributes to generate reports, cut lists, or bills of material.
- Automate common tasks like generating scenes from a set of rotations.
- Animate stuff, from drawing elements to camera position.
- ...and much more!!
What is a Ruby Plugin?
Here's a little bit of a SketchUp History lesson! In the SketchUp world, "plugin" historically meant any ruby code that could be added to SketchUp to extend SketchUp's functionality. "Scripts" and "Rubies" are other terms that mean "Plugin." You may see these terms used interchangeably. All of these terms refer to text files with a .rb (ruby) or .rbs (scrambled ruby) extension that contain Ruby programming code. Since about SketchUp 2013, SketchUp has been moving away from "Plugins" and encouraging developers to embrace "Extensions."What is a SketchUp Extension?
A SketchUp Extension is like any other Ruby script, except for the fact that you make a few extra code calls to tell SketchUp that it's an "extension." When you tell SketchUp that you are loading an extension, you can also tell it copyright information, developer information, and a description of the extension. All these data are visible to the user inside of SketchUp. Anoher benefit is that users can easily turn extensions on and off without having to fully uninstall and reinstall them. Extensions are a little more work to code, but they make it easier for end users to manage their SketchUp installation.Okay then what is a Ruby C Extension?
A Ruby C Extension is not a SketchUp Ruby APi specific term. It is a compiled library that can add functionality to Ruby. With some special compiling, you can write Ruby C Extensions that your SketchUp Extensions can interact with. If you have some intense computing that needs to be done in your extension, you might investigate writing that as a C Ruby C Extension because it will be more performant than writing the same code directly in uncompiled Ruby. A Google search for Ruby C Extension is a great place to start learning more. You can also find an example of how to compile Ruby C Extensions specificaly for us with SketchUp at our SketchUp Ruby C Extensions Examples page on Github.Do I need SketchUp Pro to run or create Ruby Extensions?
No. The full Ruby API is included in both SketchUp Make and SketchUp Pro.So how do I learn to use the Ruby API?
The Getting Started section of this website is a great place to learn the basics of how to access the Ruby API.How do I learn to write a complete Extension?
View the ruby documentation for the SketchupExtension class. Also, check out our complete Extension example in the Example Extensions section. Hello Cube walks you through everything that you need to do to make your Ruby script take advantage of the features offered to full Extensions.Where can I find SketchUp Ruby Plugins?
SketchUp maintains a Warehouse just for Extensions. Everyone that has SketchUp has access to the Extension Warehouse from inside of SketchUp. That is our favorite place for finding and distributing extensions.
The Extension Warehouse is not the only place though that offers extensions. You can also find a number of free and commercial scripts at:
Where can I find other SketchUp Ruby programmers to network with?
The official group can be found at Developer Category on our SketchUp Forum. There is also a wonderful developer community at http://www.sketchucation.com.What is the SketchUp Ruby Console?
The SketchUp Ruby Console is a small dialog that you can display using the Window > Ruby Console menu item. The Console provides direct access to SketchUp Ruby Interpreter. There is an input box where you can type Ruby commands and an output window where you can view debugging output.Advanced Questions
If I make changes to my script, how do I reload it without restarting SketchUp?
Open the SketchUp Ruby Console by selecting Window > Ruby Console from the menu. Let's say the script is called HelloWorld.rb. Simply type in "load 'HelloWorld.rb' " into the input box and SketchUp will reload and execute the script.As a plugin author, how can I prevent people from stealing my script?
You should encrypt your extension which can be done our our Digital Signature and Encryption page. Or it is also done for you when you upload your extension to the Extension Warehouse.I don't have a (Mac/PC), how can I test on both platforms?
The best practice is to test thoroughly on both platforms. You might try asking on the SketchUp Developer Forum if anyone would be willing to help you test.What are the differences between the Mac and PC when it comes to the Ruby API?
Previous to SketchUp 2017, the biggest difference between the two platforms were the WebDialogs. On the PC, the embedded browser is Internet Explorer, and on the Mac it's Safari. With 2017 SketchUp has Chromium Embedded Framework include. You should us the new HtmlDialog class to take advantage of Chromium. This makes it much simpler to acheive the same HTML results on both platforms. If you're not using WebDialogs or HtmlDialogs for anything, then you don't have to worry too much about it.Another difference is the fact that the Mac supports "MDI" (Multiple Document Interface), meaning there can be more than one SketchUp model open at a time under the same SketchUp process. This can cause Ruby scripting challenges if your code is doing something with an entity only to have the user change the active model out from under you. There's no easy answer for how to handle all of the potential problems with this... it's probably enough for you to be aware of it and be sure to test what happens when a Mac user changes the active window while your script is active.
Finally, there is a difference in the way that the Mac boots up SketchUp that you should be cautious about: there is no Sketchup.active_model when the Ruby scripts are first loaded. So if your script is making changes to the active_model at load time, it will not work on the Mac. The answer? Ensure code that references the active model is part of a UI event handler, responding to the user selecting a tool or a menu item. You can also use an AppObserver to get a callback whenever a new model is opened, at which point it's safe to talk to the active_model.
How can I detect if my plugin is running on the Mac vs. PC?
You can get OS information from Object::RUBY_PLATFORM.Here's a commonly used snippet of code:
Are there any debugging tools available for Ruby?
The most common way to debug your Ruby code is to use lots of puts() statements to print things to the Ruby console and watch what's going on, or even using Ruby's file access capabilities to write to log files.An even better option is the SketchUp Ruby Debugger that we created and host on our Github page. It takes a little more effort to setup, however it is the most comprehensive way to debug ruby inside of SketchUp. If you're really stuck on something, post your problem to the SketchUp Developer Forum. We're all happy to help.
What parts of Ruby comes with SketchUp?
SketchUp ships the Ruby Core classes as well as most of the Standard Library. Some Standard Library classes were removed because it was not possible to support them while running ruby embedded within the SketchUp application.I need a Ruby module (such as CGI) that doesn't come with SketchUp. What do I do?
The best solution would be to distribute the needed .rb files in a subdirectory that you add to the /Plugins directory when they install your script.Where can I find documentation on all of the things Ruby can do in general?
Visit the main ruby site at http://www.ruby-lang.org/.Can I use the Ruby API to generate a Bill of Materials from a model?
The short answer: yes.The long answer: a bill of materials is a complex thing that is different for each industry and user group. Since SketchUp is a surface modeler that doesn't necessarily know that the box you're drawing is, say, a piece of lumber, there could be a fair amount of work that your script would need to do to get the results that you want. But that's why it's a generic programming API: you can do whatever you want with it.
Can my plugin run off the Web?
Sort of. SketchUp's HtmlDialog class allows your plugin to open an embedded web browser inside of SketchUp and display a website (or a user interface hosted on a website.) With some javascript coding, it is possible for your website to then talk to the Ruby API (subject to certain security considerations).If my plugin works in the current version of SketchUp, will it work in earlier versions?
Not necessarily. See the release notes for the API features that have been added in each version. Also each method in the API Docs lists what version of SketchUp the method was introduced in. Make sure to only use methods available in your target SketchUp versions. If you use methods that are only around in the latest version, then expect that a certain percentage of your users will have problems. The good news is that you can detect the user's version in your plugin and react accordingly:How do I detect the version of SketchUp a user is running?
I found a bug in the API. What do I do?
You can help us best by first searching the SketchUp Developer Forum to see if your bug has already been reported in the forums. You might also want to search on Sketchucation. The SketchUp team watches and responds to bug reports on both of these forums.
If you can't find a reference to your bug, please post it along with any information you can provide that might help us reproduce your bug.
I have a feature request for the API. What do I do?
We recommend posting feature requests to the SketchUp Developers group. You might also want to make a post to the Ruby API forum on Sketchucation. There is a sticky post there specifically for API requests.How can I add my own menu item to SketchUp?
See the Menu class for detailed examples of creating your own Tool.Is Bryce a real person?
Some say yes, others disagree. Those of us who work in the SketchUp office have our own personal, deeply held beliefs on this topic. I personally think of Bryce as the Great Brycosauraus Rex. Probably everyone else in the office just thinks of Bryce as the SketchUp Core product manager who has been with the SketchUp team "forever." Bryce gained SketchUp immortatlity when his Face-Me component was used as the person inside the architectural templates in SketchUp 6. Believe what you would like about him, but one thing is certain - altering his component into hilarious situations is easily one of the greatest joys you can experience on this earth.